The application consists of an application component launcher and the following application components:
- Finan
Financial stuff. - Invoices and Properties
Basically two linked tables: invoices and the related properties. - Media
Applications related to music, movies and pictures. - Rolodex
Names, addresses and phonenumbers. - Unit Converter
An application to convert different units for speeds and distances. - Vacations
The application to organize all information about your vacations. - PCTools
A set of tools, mainly to maintain your PC. - Events
‘Important’ events in your life.
The MyWorld main class is an application launcher, which either launches the MyWorldMenuWindow, or any specific application component.
General application component design
- Windows extend JfxStage
Every window (so also the main window) extendsgoedegep.jfx.JfxStage
. - Registry
Every application has a Registry class, which contains information to be shared between classes within the application.
Standard fields:
developmentMode – If true, the application runs in development mode. In this mode e.g. extra menu items related to development may be available.
TODO: specify in which package it shall be. - Customization
A Customization combines:- a Look (colors, fonts, ..)
- AppResources (icons, …)
- ComponentFactory
A factory to create components in the right look.
- Sub-component launcher
If an application component has sub components, it has a component launcher to launch the sub components. The name of the launcher class is <component>MenuWindow. - Window title
The window title is defined via a constant WINDOW_TITLE.
If a window is operating on a file, the format of the title is:
<title> – <dirty><file-name>
Where:
<title> is the (language specific) window title, defined via WINDOW_TITLE
* <dirty> is a ‘*’ symbol if the data file is dirty, empty otherwise
* <file-name> is the name of the file being operated on.
The window title is updated by a methodupdateTitle()
. - Constructor
The first parameter is for the customization:CustomizationFx customization
- Method to create the GUI
In every ‘Window’ class (a class which extends JavaFxStage) the GUI is created by a methodcreateGUI()
. - Controls are created via ComponentFactoryFx
All controls have to be created via aComponentFactoryFx
for the specified customization.
This component factory is obtained in the constructor. - Development mode
Every application has an attribute developmentMode in its registry. By default its value is false. If true, the application will
have extra functionality for development. For example a PropertyDescriptors editor.
DevelopmentMode is active when running in Eclipse. If the current directory ends with ‘target/classes’ we assume we’re running within eclipse. - Properties
Every application has a PropertyDescriptor file, named (by convention) src/main/resources/MyProjectPropertyDescriptors.xmi.
import goedegep.appgenfx.JavaFxStage; Public class ApplicationComponentMenuWindow extends JavaFxStage { private static final Logger LOGGER = Logger.getLogger(ApplicationComponentMenuWindow.class.getName()); private static final String WINDOW_TITLE = "Window title"; private ComponentFactoryFx componentFactory; public ApplicationComponentMenuWindow(CustomizationFx customization) { super(WINDOW_TITLE, customization); componentFactory = getComponentFactory(); createGUI(); } /** * Create the GUI. */ private void createGUI() { } }
Tailoring
This is about everything that can be changed without modifying the application (the java code).
MyWorld application starts with creating a PropertyDescriptorsResource for its own PropertyDescriptors file (MyWorldPropertyDescriptors.xmi). The PropertyDescriptorsResource reads the file and provides the information as a PropertyDescriptorGroup. It also stores the properties marked as storeInitialValue in the Registry indicated by the property registryClassName of the PropertyDescriptorGroup.
The file MyWorldPropertyDescriptors.xmi contains information for the MyWorld application, but also provides the names of the property descriptor files for all other components.
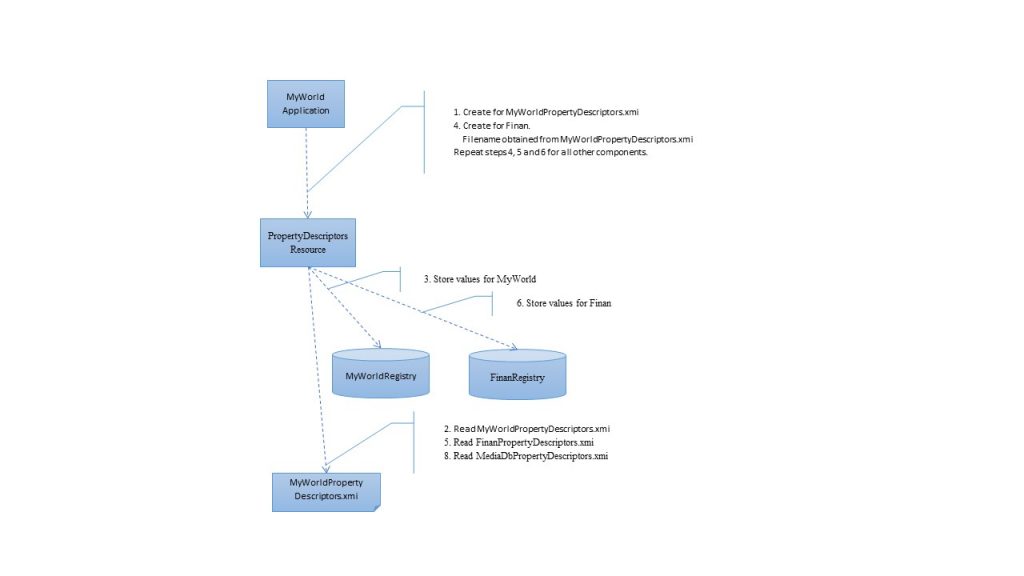
Next the MyWorldApplication creates a PropertyDescriptorsResource for each other component to be initialized.
Model Configuration
TODO: Rename to Appearance with AppearanceInfo, ModuleAppearanceInfo and Appearance.
TODO: Rename the colors to English
TODO: parentModuleLook is not needed. Just add an operation getParentModuleLook().
This model provides the data structures to describe the appearance of a module. The appearance is described by:
- the name of a class which provides images
- a set of GUI colors
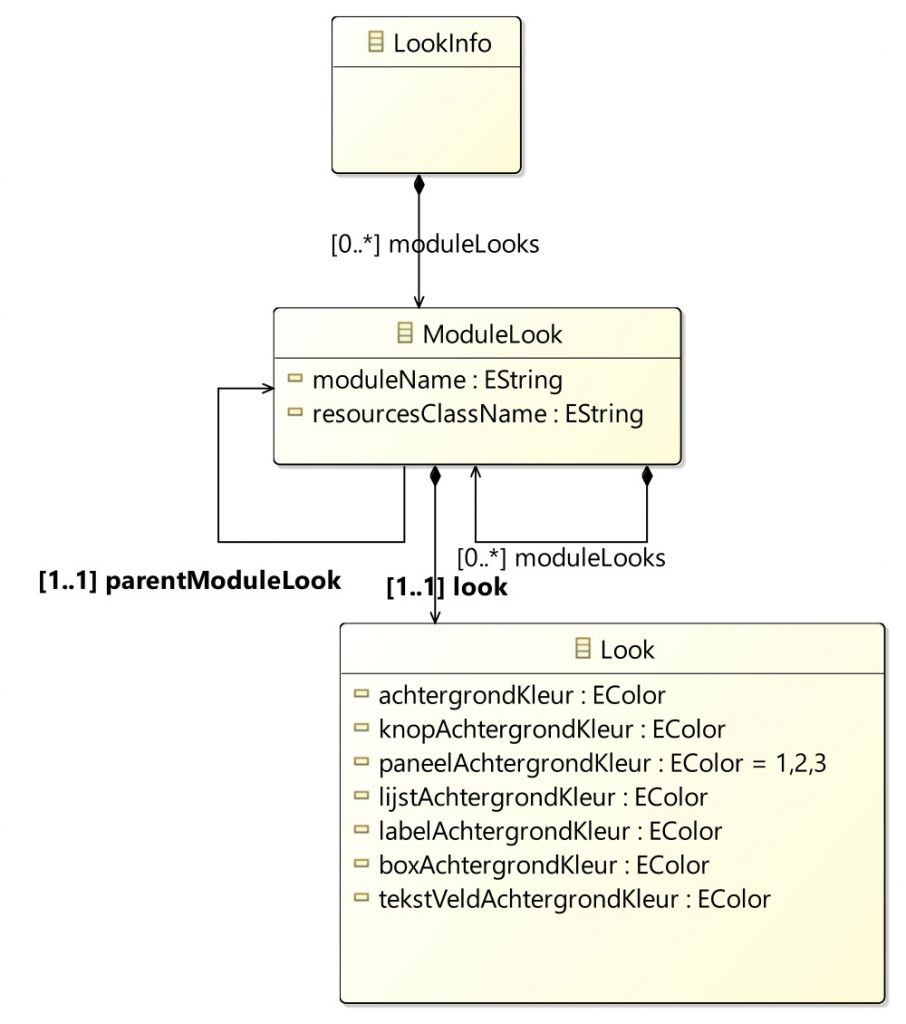
Class LookInfo
Top level class which is only a container for the ModuleLook
s.
Class ModuleLook
Describes the appearance of a (sub) module. Attributes:
- moduleName (String)
The name of the module to which this information applies. - resourcesClassName (String)
The name of a class which provides images. These are images like: the icon in the title bar, images shown on a button, and background images. - look (Look)
A reference to a set of GUI colors. - moduleLooks (list of ModuleLook)
A list of module looks for any sub modules of this module. - parentModuleLook (ModuleLook)
A reference to the parent module look.
Class Look
This class defines the look of an application. Currently it only defines colors for the GUI controls. Attributes: TODO after rename.
Class Customizations
This class is used to maintain a set of CustomizationFx
‘s, identified by their related module names.
Component MyWorld
The following diagram provides an overview of the MyWorld component.
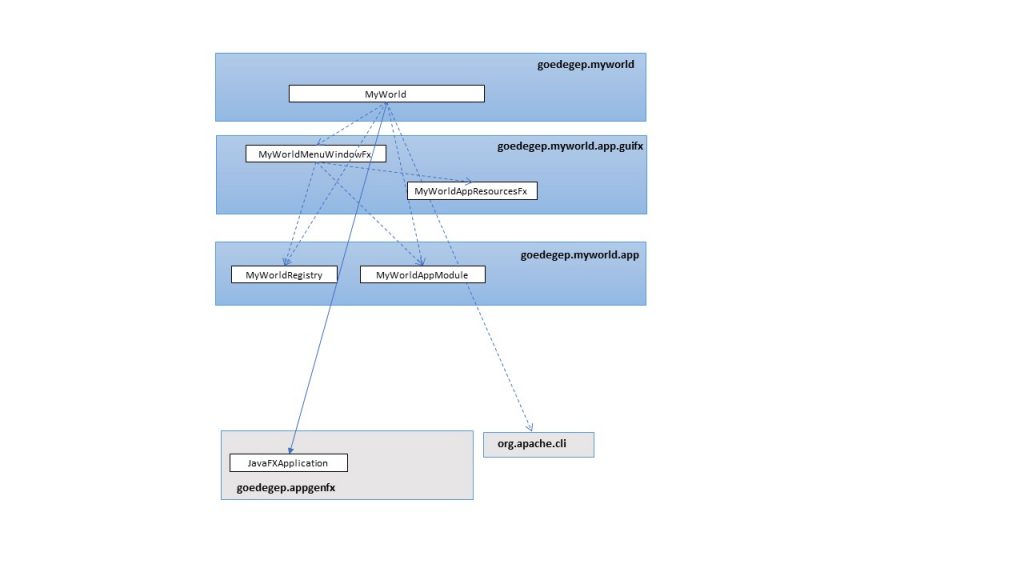
Class MyWorld
This class is the starting point of the application. It:
- Handles the command line arguments
The Apache Commons CLI library is used to parse the command line arguments.
The command line arguments provide the following information:- The main user data directory for all applications
This is passed as a parameter to the MyWorld Constructor. - The application to start
By default the MyWorldMenuWindow is started, but if an application is specified, only this application is started.
The application to start (appModule) is identified as a MyWorldAppModule.
- The main user data directory for all applications
- It determines whether the application is running in eclipse and if so, sets developmentMode to true in all relevant registries (see Development mode).
- Where applicable, command line arguments are passed on to the applicable registries.
Class MyWorldMenuWindowFx
This class provides the main window of the MyWorld application. This main window is a component launcher.
Class MyWorldAppResourcesFx
This class provides the resources (images) for the MyWorld component.
Class MyWorldRegistry
This class is the registry class for this component.
Enum MyWorldAppModule
This enum defines the modules of the application. Each module is given a name, and for each module a list of modules on which it depends is specified.